The short answer for right now is "no", but you can create a custom tool in a Toolbox by right clicking on the Toolbox > New > Script.
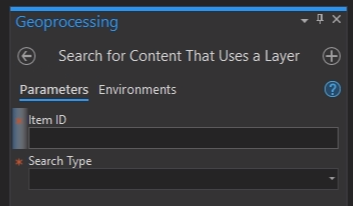
Since @jcarlson was so generous with sharing his code, I'd like to share what I did to take it a step further and set up this tool so that others in my organization who didn't have programming experience could search for items. (setup for ArcGIS Pro)
When setting up the tool parameters, I entered "Web Map" and "Web Application" in the Value List I created for that parameter. This creates a drop down in the tool and ensures there won't be any typos in the user input that would break the code.
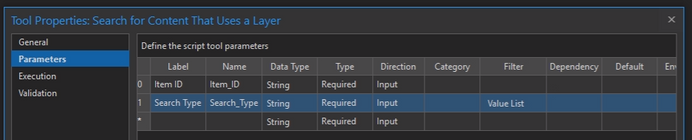
Also, if anyone reads this who is completely new to Python, I wanted to point out that when setting up tools to use within Pro or ArcMap, you use arcpy.AddMessage() to print messages to the console instead of a traditional print() statement.
And this is what the output looks like (nothing fancy) - this particular layer is only used in one web map. (side note - the time it takes to run depends on the number of items in your Organization's contents. It may run much quicker if you have fewer items to search through).
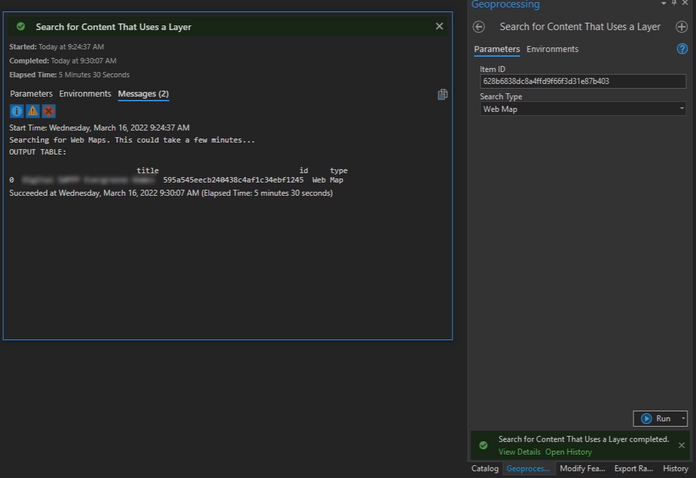
This community has helped me so much throughout the years, I like to give back when I can. I hope this helps.....
Best,
Katherine
from arcgis.gis import GIS
import pandas as pd
# Log in to portal; 'home' uses the credentials used to login within Pro
gis = GIS('home')
# Set up input parameters to use in the GUI
find_id = arcpy.GetParameterAsText(0)
search_type = arcpy.GetParameterAsText(1)
find_url = gis.content.get(find_id).url
if search_type == 'Web Map':
arcpy.AddMessage("Searching for Web Maps. This could take a few minutes...")
# Pull list of all web maps in portal
webmaps = gis.content.search('', item_type='Web Map', max_items=-1)
# Return subset of map IDs which contain the service URL we're looking for
matches = [m.id for m in webmaps if str(m.get_data()).find(find_url) > -1]
# Create empty list to populate with results
map_list = []
# Check each web map for matches
for w in webmaps:
try:
# Get the JSON as a string
wdata2 = str(w.get_data())
criteria = [
wdata2.find(find_url) > -1, # Check if URL is directly referenced
any([wdata2.find(i) > -1 for i in matches]) # Check if any matching maps are in app
]
# If layer is referenced directly or indirectly, append map to list
if any(criteria):
map_list.append(w)
# Some apps don't have data, so we'll just skip them if they throw a TypeError
except:
continue
output = pd.DataFrame([{'title': m.title, 'id': m.id, 'type': m.type} for m in map_list])
arcpy.AddMessage(f"OUTPUT TABLE: \n \n {output}")
if search_type == 'Web Application':
arcpy.AddMessage("Searching for Web Applications. This could take a few minutes...")
# Pull list of all web apps in portal
webapps = gis.content.search('', item_type='Application', max_items=-1)
# Create empty list to populate with results
app_list = []
# Return subset of map IDs which contain the service URL we're looking for
matches = [a.id for a in webapps if str(a.get_data()).find(find_url) > -1]
# Check each web app for matches
for w in webapps:
try:
# Get the JSON as a string
wdata = str(w.get_data())
criteria = [
wdata.find(find_url) > -1, # Check if URL is directly referenced
any([wdata.find(i) > -1 for i in matches]) # Check if any matching maps are in app
]
# If layer is referenced directly or indirectly, append app to list
if any(criteria):
app_list.append(w)
# Some apps don't have data, so we'll just skip them if they throw a TypeError
except:
continue
output = pd.DataFrame([{'title':a.title, 'id':a.id, 'type':a.type} for a in app_list])
arcpy.AddMessage(f"OUTPUT TABLE: \n \n {output}")
Best,
Katie
“The goal is not simply to ‘work hard, play hard.’ The goal is to make our work and our play indistinguishable.”
- Simon Sinek