@Katie_Clark, you guess right: you can do this with the Python API!
The text version:
- For a given layer's URL
- Find all maps that reference that layer
- Fina all apps that reference those maps or the layers directly
The tricky part is # 3. An app can reference a layer directly in one of its widgets, like the Search widget. Other apps, like Experience Builder and Dashboards, can also reference layers directly without going through a map. Further complicating this is that certain apps will make direct references to a layer using their ID, rather than their URL.
To make sure our search is comprehensive we can't just search for the apps that reference the maps; we'll have to search for the layers directly as well. It gets a bit convoluted, but it works!
The code:
from arcgis.gis import GIS
import pandas as pd
# Log in to portal; prompts for PW automatically
gis = GIS('your-portal-url', 'username')
# Layer ID to search for and its URL
find_id = 'a405b06ae8e24f94a2768a4581b79e73'
find_url = gis.content.get(find_id).url
# Pull list of all web maps in portal
webmaps = gis.content.search('', item_type='Web Map', max_items=-1)
# Return subset of map IDs which contain the service URL we're looking for
matches = [m.id for m in webmaps if str(m.get_data()).find(find_url) > -1]
# Pull list of all web apps in portal
webapps = gis.content.search('', item_type='Application', max_items=-1)
# Create empty list to populate with results
app_list = []
# Check each web app for matches
for w in webapps:
try:
# Get the JSON as a string
wdata = str(w.get_data())
criteria = [
wdata.find(find_url) > -1, # Check if URL is directly referenced
any([wdata.find(i) > -1 for i in matches]) # Check if any matching maps are in app
]
# If layer is referenced directly or indirectly, append app to list
if any(criteria):
app_list.append(w)
# Some apps don't have data, so we'll just skip them if they throw a TypeError
except:
continue
pd.DataFrame([{'title':a.title, 'id':a.id, 'type':a.type} for a in app_list])
The output DataFrame will be any apps that reference your layer:
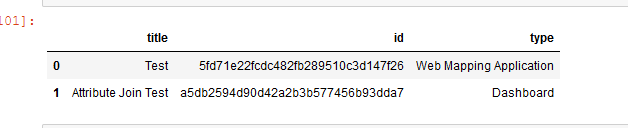
You can easily amend the final line of the expression to include additional details about the items.
- Josh Carlson
Kendall County GIS