I just downloaded the sample data, and ran my code from the Draw a Line to the nearest Polygon thread, and it ran without any errors and did exactly what you wanted. I did have to increase the dist value since you are using State Plane, and the gaps between the lines and river banks are greater than 100 feet.
Here is the code that worked for me, using your 2 shape files (load the shape files into ArcMap first and run from interactive Python window):
>>> import math
>>> dist = 500
>>>
>>> fc_line = "Pointoline"
>>> fc_poly = "RB_23"
>>> with arcpy.da.SearchCursor(fc_poly, "SHAPE@") as scur:
... for poly, in scur:
... boundary = poly.boundary()
... arcpy.SelectLayerByLocation_management(fc_line, "WITHIN", poly)
... with arcpy.da.UpdateCursor(fc_line, "SHAPE@") as ucur:
... for line, in ucur:
... arr, = line.getPart()
... SR = line.spatialReference
...
... p1, p2 = arr[:2]
... angle = math.atan2(p2.Y - p1.Y, p2.X - p1.X)
... p = arcpy.Point(p1.X - dist * math.cos(angle),
... p1.Y - dist * math.sin(angle))
... arr.insert(0, p)
...
... pn1, pn = arr[-2:]
... angle = math.atan2(pn.Y - pn1.Y, pn.X - pn1.X)
... p = arcpy.Point(pn.X + dist * math.cos(angle),
... pn.Y + dist * math.sin(angle))
... arr.append(p)
...
... line = arcpy.Polyline(arr, SR)
... line = line.cut(boundary)[1]
... ucur.updateRow([line])
...
>>>
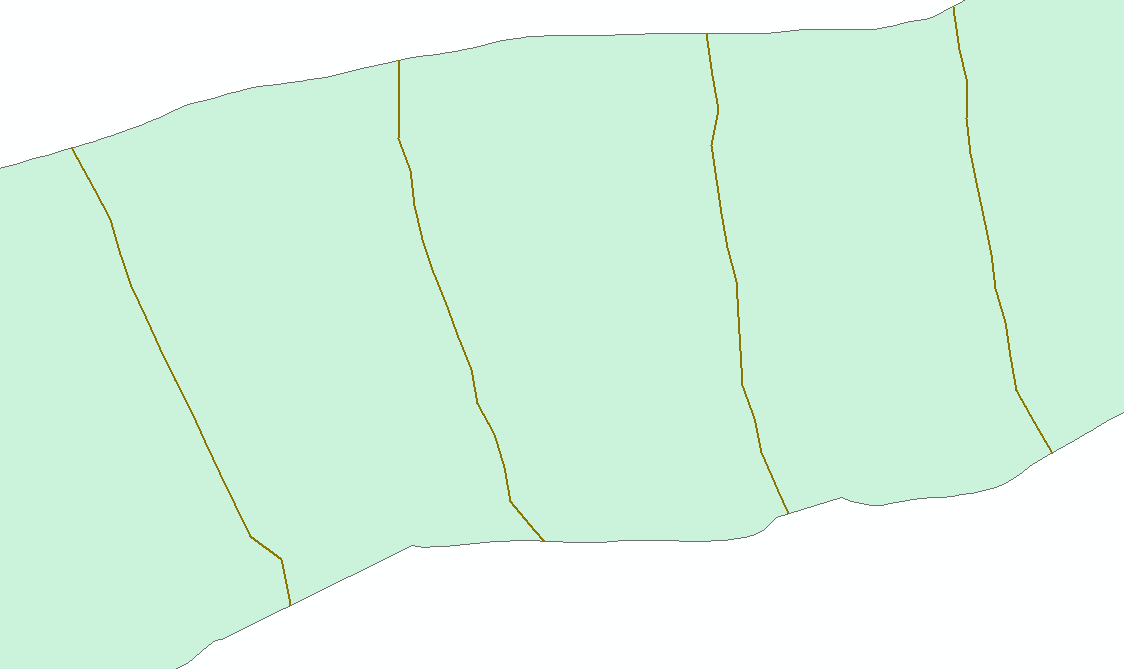