Well this doesn't quite do what you want, but makes a condensed version of the union output with each poly now recording the count of overlaps and a list of the original polygons that went into them.
import sys, os, arcpy
from arcpy import env
HomeDir = r"path to directory"
scratchFgdb = os.path.join(HomeDir, "scratch.gdb")
fc = "Input_Union"
env.workspace = scratchFgdb
env.overwriteOutput = True
desc = arcpy.Describe(fc)
sr = desc.spatialReference
poly_dict = {}
with arcpy.da.SearchCursor(fc, ["ORIG_FID", "SHAPE@", "SHAPE@XY"]) as Cur:
for row in Cur:
origFid = row[0]
geom = row[01]
cent = row[2]
x = round(cent[0], 1)
y = round(cent[1], 1)
Key = "{:.1f}/{:.1f}".format(x, y)
if Key in poly_dict:
poly_dict[Key][1] += 1
poly_dict[Key][2].append(origFid)
else:
poly_dict[Key] = [geom, 1, [origFid]]
outFc = "tmpOverlapPoly"
arcpy.CreateFeatureclass_management("in_memory", outFc, "POLYGON", "", "", "", sr)
arcpy.AddField_management(os.path.join("in_memory", outFc), "Key", "TEXT", "", "", 50)
arcpy.AddField_management(os.path.join("in_memory", outFc), "COUNT", "SHORT")
arcpy.AddField_management(os.path.join("in_memory", outFc), "FidList", "TEXT", "", "", 255)
inCur = arcpy.da.InsertCursor(os.path.join("in_memory", outFc), ["Key", "COUNT", "FidList", "SHAPE@"])
for k, v in poly_dict.items():
Key = k
geom = v[0]
cnt = v[1]
fids = v[2]
fidStr = ",".join(str(f) for f in fids)
data = [Key, cnt, fidStr, geom]
inCur.insertRow(data)
del inCur
arcpy.CopyFeatures_management(os.path.join("in_memory", outFc), outFc)
Looks like this :
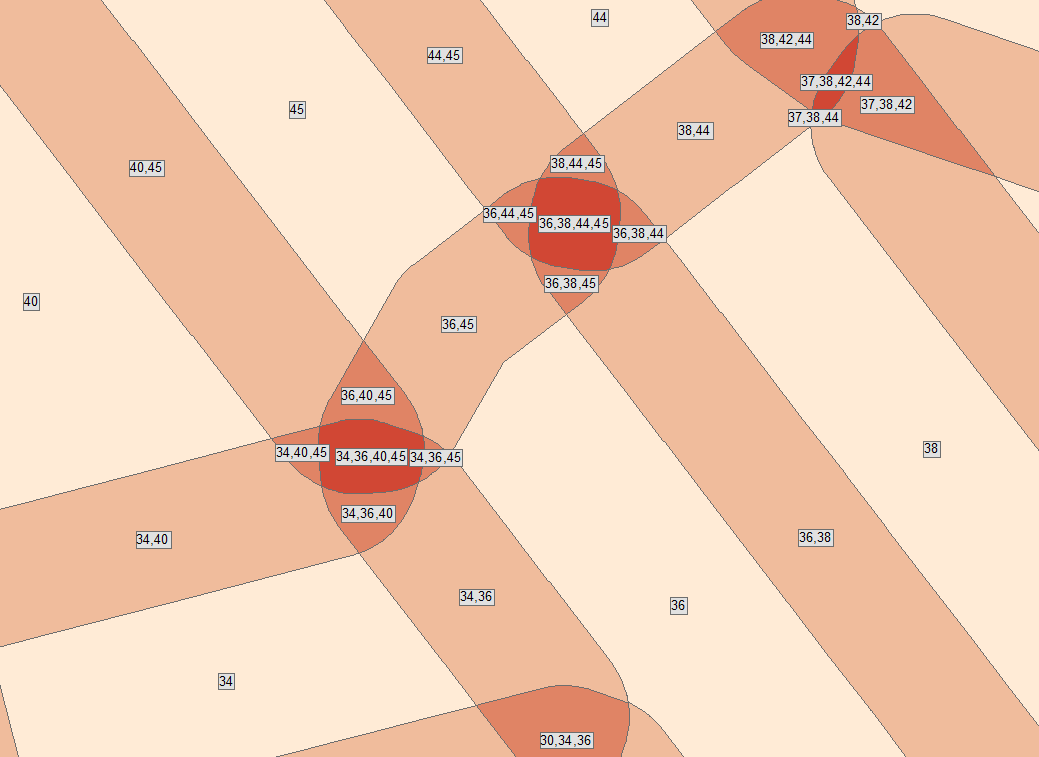