Hi Joshua and Rhett Zufelt, sorry I have had a really busy day in school. Thank you for looking over at my question.
To clarify some more of what it is I am trying to do.
I have a feature class, named TWP_Messages which is a spatial join of GPS points and the ATS grid system in Alberta.
I have sorted this feature class by a count field for the amount of GPS messages in each township. I then want to take the top result of that join and export it into a GeoJSON file which I will then use, incorporated with a script I have written to download satellite imagery from Planet Labs. I however only want to do this one township (1 cell of the ATS grid) at a time so not to be overloaded.
To achieve this I was hoping to find a way that a search cursor, or similar will take the first area from the spatial join, but as it exports this area it writes the area name in to a text file. I then ideally would like that text file to be used as a check list so when I next run the script it will then look at the text file, know that it has taken the top ranked area from the sorted join feature class and then move on to the next area in the list.
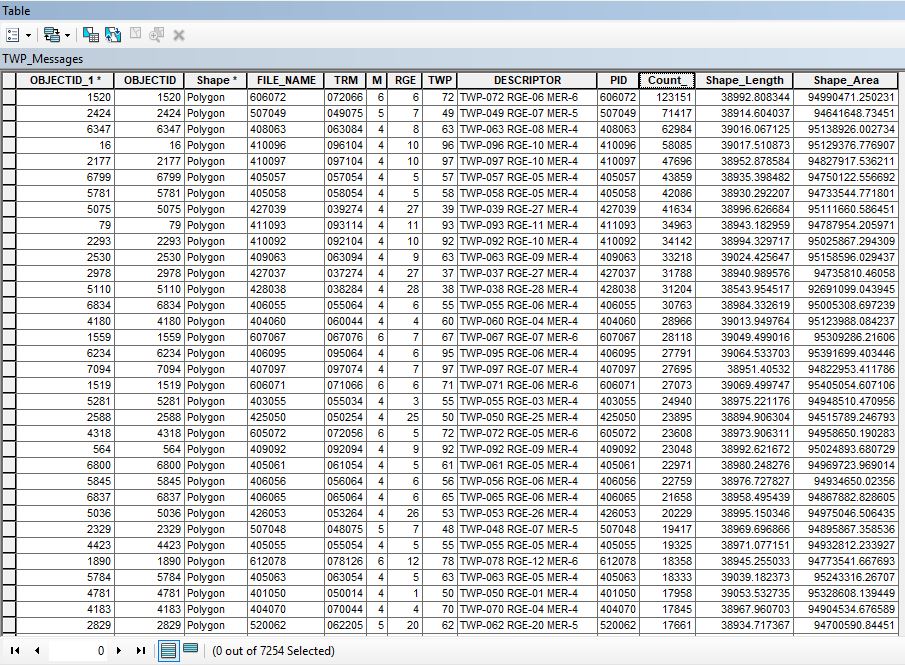
So in this feature class the first time it runs it will select the row for object ID 1520, the second time the script runs I would like for it to be able to check which areas have already been selected, so the first row in the table, ignore that and then move on to the second row so it can be exported.
I hope that is easier to understand. I am sure it can be done but I cant seem to find a similar instance where something similar has been done before.
Again, thank you for all your help. I hope I have described the situation better.