OK, so if you use a snippet like this one:
def main():
import arcpy
fc_pnt = r"C:\Forum\LongestFlowPath\data.gdb\centroid"
fc_lin = r"C:\Forum\LongestFlowPath\data.gdb\flowpath"
# get the first point and first polyline
point = [r[0] for r in arcpy.da.SearchCursor(fc_pnt, ("SHAPE@"))][0]
polyline = [r[0] for r in arcpy.da.SearchCursor(fc_lin, ("SHAPE@"))][0]
# get the tuple
tpl = polyline.queryPointAndDistance(point, False)
print tpl
if __name__ == '__main__':
main()
It will return:
(<PointGeometry object at 0x2233e70[0x2233480]>, 590.9600881822321, 190.0152317659558, True)
Which means: (point on line, distance from start, distance to line, bool right side of line), which makes sense, since it is the same as ArcObjects does.
To expand the code to extract the longest flow path you can do this:
def main():
import arcpy
fc_pnt = r"C:\Forum\LongestFlowPath\data.gdb\centroid"
fc_lin = r"C:\Forum\LongestFlowPath\data.gdb\flowpath"
# get the first point and first polyline
point = [r[0] for r in arcpy.da.SearchCursor(fc_pnt, ("SHAPE@"))][0]
polyline = [r[0] for r in arcpy.da.SearchCursor(fc_lin, ("SHAPE@"))][0]
# get the tuple
tpl = polyline.queryPointAndDistance(point, False)
# to extract the part from the point on the line to the end:
lfp = polyline.segmentAlongLine(tpl[1], polyline.length, False)
arcpy.CopyFeatures_management([lfp], r"C:\Forum\LongestFlowPath\data.gdb\lfp")
# point on line
arcpy.CopyFeatures_management([tpl[0]], r"C:\Forum\LongestFlowPath\data.gdb\projected_pnt")
if __name__ == '__main__':
main()
This is what it looks like:
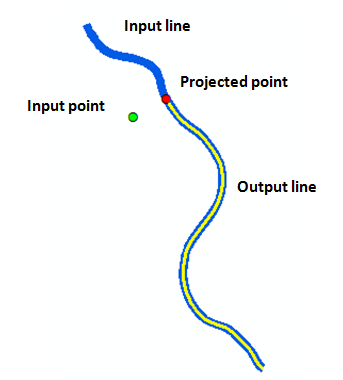