Thomas Marshall
Since I can't attach through a comment, below are the Sql functions, and some notes to be aware of...
- These functions calculate longitude and latitude for Lambert Conformal Conic 2-parallel projections (as this is what our production features are stored in (wkid: 6463 --- NAD 1983 (2011) Iowa State Plane North))
- Note lines 59 through 76 --- you will need to provide the values for your coordinate system
- You can find these values within the XY Coordinate System properties of a feature class
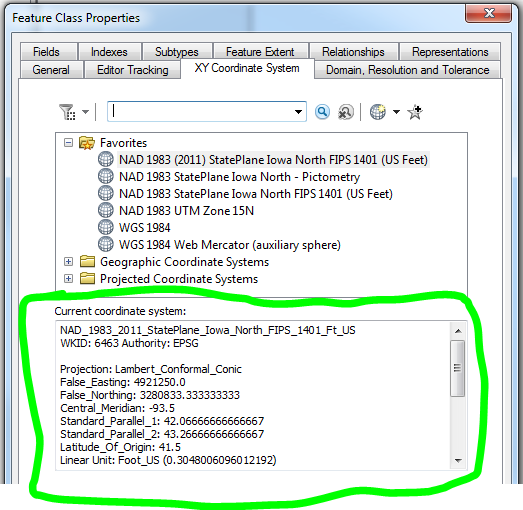
- I do this by storing the values in a table and call them through a SELECT statement, that way the values are stored in one place (example below)

Longitude Calculation
USE [**DatabaseName**]
GO
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
CREATE FUNCTION [dbo].[get_longitude]
(
@x numeric(24,12),
@y numeric(24,12)
)
RETURNS numeric(24,12)
AS
BEGIN
DECLARE
@pi numeric(24,12),
@fx numeric(24,12),
@fy numeric(24,12),
@cmD numeric(24,12),
@cmR numeric(24,12),
@sp1D numeric(24,12),
@sp1R numeric(24,12),
@sp2D numeric(24,12),
@sp2R numeric(24,12),
@loD numeric(24,12),
@loR numeric(24,12),
@smjrM numeric(24,12),
@smjrF numeric(24,12),
@smnrM numeric(24,12),
@smnrF numeric(24,12),
@flat numeric(24,12),
@iflat numeric(24,12),
@e numeric(24,12),
@m1 numeric(24,12),
@m2 numeric(24,12),
@t1 numeric(24,12),
@t2 numeric(24,12),
@tf numeric(24,12),
@n numeric(24,12),
@F numeric(24,12),
@rf numeric(24,12),
@rz numeric(24,12),
@tz numeric(24,12),
@zz numeric(24,12),
@lonR numeric(24,12),
@lonD numeric(24,12)
SET @pi = pi()
SET @fx =
SET @fy =
SET @loD =
SET @loR = @loD * @pi / 180
SET @cmD =
SET @cmR = @cmD * @pi / 180
SET @sp1D =
SET @sp1R = @sp1D * @pi / 180
SET @sp2D =
SET @sp2R = @sp2D * @pi / 180
SET @smjrM =
SET @smjrF = @smjrM * 3.2808333
SET @smnrM =
SET @smnrF = @smnrM * 3.2808333
SET @iflat = @smjrM / (@smjrM - @smnrM)
SET @flat = 1 / @iflat
SET @e = SQRT(2 * @flat - SQUARE(@flat))
SET @m1 = COS(@sp1R) / POWER((1 - SQUARE(@e) * SQUARE(SIN(@sp1R))), 0.5)
SET @m2 = COS(@sp2R) / POWER((1 - SQUARE(@e) * SQUARE(SIN(@sp2R))), 0.5)
SET @t1 = TAN((@pi / 4) - (@sp1R / 2)) / POWER((1 - (@e * SIN(@sp1R))) / (1 + (@e * SIN(@sp1R))), (@e / 2))
SET @t2 = TAN((@pi / 4) - (@sp2R / 2)) / POWER((1 - (@e * SIN(@sp2R))) / (1 + (@e * SIN(@sp2R))), (@e / 2))
SET @tf = TAN((@pi / 4) - (@loR / 2)) / POWER((1 - (@e * SIN(@loR))) / (1 + (@e * SIN(@loR))), (@e / 2))
SET @n = (LOG(@m1) - LOG(@m2)) / (LOG(@t1) - LOG(@t2))
SET @F = @m1 / (@n * POWER(@t1, @n))
SET @rf = @smjrF * @F * POWER(@tf, @n)
SET @rz = POWER(POWER((@x - @fx), 2) + POWER((@rf - (@y - @fy)), 2), 0.5)
SET @tz = POWER((@rz / (@smjrF * @F)), (1 / @n))
SET @zz = ATAN((@x - @fx) / (@rf - (@y - @fy)))
SET @lonR = ((@zz / @n) + @cmR)
SET @lonD = (@lonR * 180) / @pi
RETURN @lonD;
END
Latitude Calculation
USE [**DatabaseName**]
GO
SET ANSI_NULLS ON
GO
SET QUOTED_IDENTIFIER ON
GO
CREATE FUNCTION [dbo].[get_latitude]
(
@x numeric(24,12),
@y numeric(24,12)
)
RETURNS numeric(24,12)
AS
BEGIN
DECLARE
@pi numeric(24,12),
@fx numeric(24,12),
@fy numeric(24,12),
@cmD numeric(24,12),
@cmR numeric(24,12),
@sp1D numeric(24,12),
@sp1R numeric(24,12),
@sp2D numeric(24,12),
@sp2R numeric(24,12),
@loD numeric(24,12),
@loR numeric(24,12),
@smjrM numeric(24,12),
@smjrF numeric(24,12),
@smnrM numeric(24,12),
@smnrF numeric(24,12),
@flat numeric(24,12),
@iflat numeric(24,12),
@e numeric(24,12),
@m1 numeric(24,12),
@m2 numeric(24,12),
@t1 numeric(24,12),
@t2 numeric(24,12),
@tf numeric(24,12),
@n numeric(24,12),
@F numeric(24,12),
@rf numeric(24,12),
@rz numeric(24,12),
@tz numeric(24,12),
@zz numeric(24,12),
@latTR numeric(24,12),
@lat1R numeric(24,12),
@lat2R numeric(24,12),
@lat3R numeric(24,12),
@lat4R numeric(24,12),
@latFR numeric(24,12),
@latTD numeric(24,12),
@lat1D numeric(24,12),
@lat2D numeric(24,12),
@lat3D numeric(24,12),
@lat4D numeric(24,12),
@latFD numeric(24,12)
SET @pi = pi()
SET @fx =
SET @fy =
SET @loD =
SET @loR = @loD * @pi / 180
SET @cmD =
SET @cmR = @cmD * @pi / 180
SET @sp1D =
SET @sp1R = @sp1D * @pi / 180
SET @sp2D =
SET @sp2R = @sp2D * @pi / 180
SET @smjrM =
SET @smjrF = @smjrM * 3.2808333
SET @smnrM =
SET @smnrF = @smnrM * 3.2808333
SET @iflat = @smjrM / (@smjrM - @smnrM)
SET @flat = 1 / @iflat
SET @e = SQRT(2 * @flat - SQUARE(@flat))
SET @m1 = COS(@sp1R) / POWER((1 - SQUARE(@e) * SQUARE(SIN(@sp1R))), 0.5)
SET @m2 = COS(@sp2R) / POWER((1 - SQUARE(@e) * SQUARE(SIN(@sp2R))), 0.5)
SET @t1 = TAN((@pi / 4) - (@sp1R / 2)) / POWER((1 - (@e * SIN(@sp1R))) / (1 + (@e * SIN(@sp1R))), (@e / 2))
SET @t2 = TAN((@pi / 4) - (@sp2R / 2)) / POWER((1 - (@e * SIN(@sp2R))) / (1 + (@e * SIN(@sp2R))), (@e / 2))
SET @tf = TAN((@pi / 4) - (@loR / 2)) / POWER((1 - (@e * SIN(@loR))) / (1 + (@e * SIN(@loR))), (@e / 2))
SET @n = (LOG(@m1) - LOG(@m2)) / (LOG(@t1) - LOG(@t2))
SET @F = @m1 / (@n * POWER(@t1, @n))
SET @rf = @smjrF * @F * POWER(@tf, @n)
SET @rz = POWER(POWER((@x - @fx), 2) + POWER((@rf - (@y - @fy)), 2), 0.5)
SET @tz = POWER((@rz / (@smjrF * @F)), (1 / @n))
SET @zz = ATAN((@x - @fx) / (@rf - (@y - @fy)))
SET @latTR = (@pi / 2) - (2 * ATAN(@tz))
SET @lat1R = (@pi / 2) - (2 * ATAN((@tz * POWER((1 - (@e * SIN(@latTR))) / (1 + (@e * SIN(@latTR))), (@e / 2)))))
SET @lat2R = (@pi / 2) - (2 * ATAN((@tz * POWER((1 - (@e * SIN(@lat1R))) / (1 + (@e * SIN(@lat1R))), (@e / 2)))))
SET @lat3R = (@pi / 2) - (2 * ATAN((@tz * POWER((1 - (@e * SIN(@lat2R))) / (1 + (@e * SIN(@lat2R))), (@e / 2)))))
SET @lat4R = (@pi / 2) - (2 * ATAN((@tz * POWER((1 - (@e * SIN(@lat3R))) / (1 + (@e * SIN(@lat3R))), (@e / 2)))))
SET @latFR = (@pi / 2) - (2 * ATAN((@tz * POWER((1 - (@e * SIN(@lat4R))) / (1 + (@e * SIN(@lat4R))), (@e / 2)))))
SET @latTD = (@latTR * 180) / @pi
SET @lat1D = (@lat1R * 180) / @pi
SET @lat2D = (@lat2R * 180) / @pi
SET @lat3D = (@lat3R * 180) / @pi
SET @lat4D = (@lat4R * 180) / @pi
SET @latFD = (@latFR * 180) / @pi
RETURN @latFD;
END