The code below seems to be working...
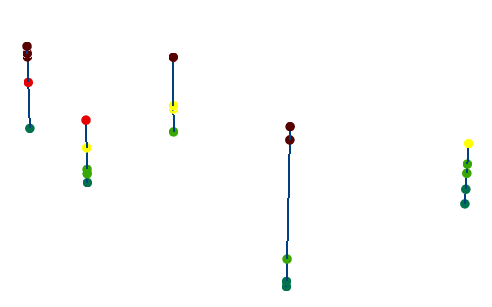
def main():
import arcpy
import os
arcpy.env.overwriteOutput = True
fc_pnt = r"D:\Xander\GeoNet\3Dpoints_3Dlines\gdb\test.gdb\points3D_v02"
fc_z = r"D:\Xander\GeoNet\3Dpoints_3Dlines\gdb\test.gdb\polylineZ"
fld_lineid = "LineID"
fld_order = "Elevation"
# create a new fc Z enabled, but no M values
fc_ws, fc_name = os.path.split(fc_z)
arcpy.CreateFeatureclass_management(fc_ws, fc_name, "POLYLINE", fc_pnt, "DISABLED", "ENABLED", fc_pnt)
# write the geometries to the new fc
sr = arcpy.Describe(fc_pnt).spatialReference
# flds_in = ("SHAPE@", fld_lineid, fld_order)
fld_shp_in = arcpy.Describe(fc_pnt).shapeFieldName
sort_flds = "{0} A; {1} A".format(fld_lineid, fld_order)
flds_in = "{0}; {1}; {2}".format(fld_shp_in, fld_lineid, fld_order)
flds_out = ("SHAPE@", fld_lineid)
lineid = "NotSet"
lst_poly_z = []
with arcpy.da.InsertCursor(fc_z, flds_out) as curs_out:
curs = arcpy.SearchCursor(fc_pnt, fields=flds_in, sort_fields=sort_flds)
for row in curs:
pnt = row.getValue(fld_shp_in)
prev_lineid = lineid
lineid = row.getValue(fld_lineid)
if prev_lineid == lineid:
# add point to line
lst_poly_z.append(pnt.firstPoint)
else:
# write previous line and start new one
if len(lst_poly_z) > 1:
polyline_z = arcpy.Polyline(arcpy.Array(lst_poly_z), sr, True, False)
row_out = [polyline_z, prev_lineid]
curs_out.insertRow(tuple(row_out))
lst_poly_z = []
lst_poly_z.append(pnt.firstPoint)
# write last polyline
if len(lst_poly_z) > 1:
polyline_z = arcpy.Polyline(arcpy.Array(lst_poly_z), sr, True, False)
row_out = [polyline_z, lineid]
curs_out.insertRow(tuple(row_out))
del curs, row
if __name__ == '__main__':
main()
- line 7 and 8 hold the paths to input 3D points and output 3D lines
- line 10 holds the name of the input field that identifies the lines (for each unique value in this field points will be combined into a single line)
- line 11 holds the name of the input field that provides the order of the points when creating the line (I used the same Z value)