Here's my problem, hope anybody can get me on the way...
I need to remove the "caps" of long polygon(road) features. The caps are created by boundary lines. Each line can slice the same polygon multiple times. After converting the line to points I'm able to select the points touching the polygon. But the selection can contain multiple start-end-points for each slice.
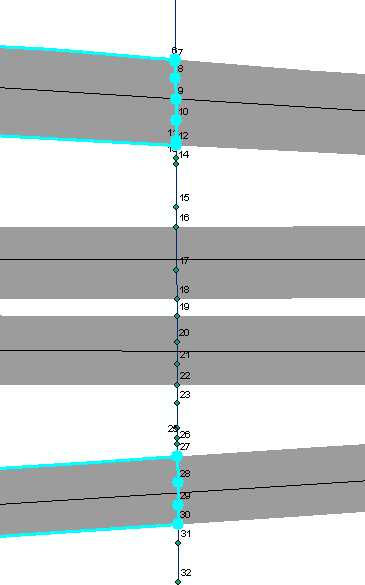
Here's the array of selected points.
[
(6, [163320.90700000152, 379580.6550000012])
(7, [163320.90900000185, 379580.34699999914])
(8, [163320.92320000008, 379577.6838000007])
(9, [163320.941300001, 379574.2791999988])
(10, [163320.95899999887, 379570.9439999983])
(11, [163320.97700000182, 379567.63800000027])
(12, [163320.98000000045, 379567.12000000104])
(27, [163321.24599999934, 379517.67599999905])
(28, [163321.26900000125, 379513.493999999])
(29, [163321.28799999878, 379509.9620000012])
(30, [163321.3040000014, 379506.8920000009])
]
And this is my goal output-array. I need these corners!
[
(6, [163320.90700000152, 379580.6550000012])
(12, [163320.98000000045, 379567.12000000104])
(27, [163321.24599999934, 379517.67599999905])
(30, [163321.3040000014, 379506.8920000009])
]
At first I tried to use the searchcursor, but i was unable to identify the next-id in a loop to see if it's ID is just+1. So my thought is to use the numpy array, but i'm still on the ground...
How do I loop this numpy array or mayby use some smart numpy function to remove unwanted rows from the array?
This is the code that created the array:
tmppt_lay = arcpy.MakeFeatureLayer_management(tmppt, "tmppoints_lay", "", "", "OBJECTID OBJECTID VISIBLE NONE;SHAPE SHAPE VISIBLE NONE;ORIG_FID ORIG_FID VISIBLE NONE")
tmppt_sel = arcpy.SelectLayerByLocation_management(tmppt_lay, "INTERSECT", bit2, "0,02 Meters", "NEW_SELECTION")
#now loop these selected points and identify future split-points
srows = arcpy.SearchCursor(tmppt_sel)
sdesc = arcpy.Describe(tmppt_sel)
selectionCount = len(sdesc.fidset.split(";"))
arcpy.AddMessage(" selected points: " + str( selectionCount))
#for srow in srows:
#got stuck here!
#try the numpy way..
sArr = arcpy.da.FeatureClassToNumPyArray (tmppt_sel, ["OID@","SHAPE@XY"], "#", rd)
oArr = arcpy.Array()
cnt = 0
for oi, xy in sArr:
if cnt==0: #this is always the startpoint
arcpy.AddMessage("start point in: " + str(loid) + ": id: " + str(oi) + ", " + str(xy))
elif (cnt==selectionCount-1): #this is always the endpoint
arcpy.AddMessage("end point in: " + str(loid) + ": id: " + str(oi) + ", " + str(xy))
else: #here i need to findout if the next point has an ID 1 larger thn the current
arcpy.AddMessage("counter + 1: " + str(int(cnt+1)))
nextcnt = cnt+1
nextrow = sArr[nextcnt]) # this does NOT work
#nextid = nextrow[0]
#arcpy.AddMessage("nextid: " + str(nextid))
cnt+=1
Het bericht is bewerkt door: Bart van der Wolf
Added a loop for the array, but stuck on the same point as with using the searchcursor.