Working with customers I've had several use cases to use the GeoEvent Admin API. For example, a GeoEvent input may be using a URL that contains an API key. This key may expire after a number of days and needs to be recreated. Instead of manually editing the GeoEvent input and updating the API key, you can use the GeoEvent Admin API to do this automatically via a Python script. You can access the GeoEvent Admin API by going to the following URL:
https://<GeoEvent Server Full Qualified Domain Name>:6143/geoevent/admin/api/index.html
This will provide a list of all supported operations and examples on how to use them. In this example, I will show how you can update the URL in a GeoEvent Input. The GeoEvent Input is a Poll an External Website for GeoJSON.
1. First you will need to get the Name of the Input. This is a GUID and can be found a couple places. If you click on the GeoEvent Input, the URL will contain the GUID after the name parameter:
https://geoevent.esri.com:6143/geoevent/manager/input.html?name=125626c5-7f42-4075-8e3b-20903982243a&type=esri-external-geojson-poll&mode=inbound&running=STARTED&clone=false
You can also obtain the input name by going to the GeoEvent REST Services Directory (https://geoevent.esri.com:6143/geoevent/rest/😞
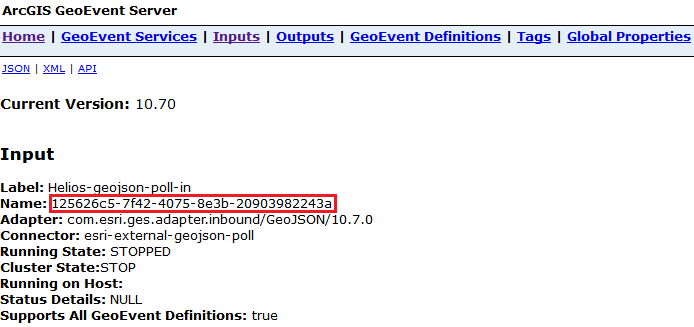
2. Once you have this, you execute the following python script after updating the # Variables:
import requests, json
requests.packages.urllib3.disable_warnings()
geoeventServer = 'geoevent.esri.com'
username = "siteadmin"
password = "******"
geoeventInput = '125626c5-7f42-4075-8e3b-20903982243a'
newURL = 'https://api.helios.earth/v1/cameras?access_token=eyJ0eXAiOiJKV1QiLCJhbGciOiJIUz'
tokenURL = 'https://{0}:6443/arcgis/tokens/'.format(geoeventServer)
params = {'f': 'pjson', 'username': username, 'password': password, 'referer': 'https://{0}:6143/geoevent/admin'.format(geoeventServer)}
r = requests.post(tokenURL, data = params, verify=False)
response = json.loads(r.content)
token = response['token']
header = {'Content-Type':'application/json', 'GeoEventAuthorization':token}
putURL = 'https://{0}:6143/geoevent/admin/input/'.format(geoeventServer)
inputURL = putURL + geoeventInput
r = requests.get(inputURL, headers=header, verify=False)
response = json.loads(r.content)
response['transport']['properties'][3]['value'] = newURL
r = requests.put(putURL, data=json.dumps(response), headers=header, verify=False)
if r.status_code == 200:
print("Successfully updated input")
else:
print("Error occurred updating input. Error: {0}".format(r.status_code))
Variables explained:
Variable | Explanation |
---|
geoeventServer | Fully qualified domain name of GeoEvent Server |
username | Primary Site Administrator username |
password | Primary Site Administrator password |
geoeventInput | GUID for GeoEvent input |
newURL | the new URL the GeoEvent input will be updated to |
Note: typically there would be additional code within this script to generate the new access_token in the newURL variable.
The # Genereate Token portion of code will generate an ArcGIS Server token using the credentials specified. Note: This is the ArcGIS Server instance on the GeoEvent server.
tokenURL = 'https://{0}:6443/arcgis/tokens/'.format(geoeventServer)
params = {'f': 'pjson', 'username': username, 'password': password, 'referer': 'https://{0}:6143/geoevent/admin'.format(geoeventServer)}
r = requests.post(tokenURL, data = params, verify=False)
response = json.loads(r.content)
token = response['token']
After the token is generated, it is specified in an HTTP header: GeoEventAuthorization: token.

A get request is made using the GeoEvent input URL. This will return JSON, which you can update by specifying the appropriate JSON object, i.e.:
response['transport']['properties'][3]['value'] = newURL
Finally, a put request is made with the updated JSON to update the Input's URL.
r = requests.put(putURL, data=json.dumps(response), headers=header, verify=False)
if r.status_code == 200:
print("Successfully updated input")
else:
print("Error occurred updating input. Error: {0}".format(r.status_code))
The script could then be executed by Windows Task Scheduler on a given interval (i.e. once every x days). The input URL would update and you would no longer have to worry about the access token expiring.